React is a popular open-source JavaScript library used for building user interfaces, especially for single-page applications where you need to dynamically update the content without reloading the entire page. Famous websites built by React includes Facebook, Instagram, WhatsApp Web, Netflix, Airbnb, Dropbox, New York Times, Trello, Spotify, Tesla, to name but a few.
Simple Hello World React website
Here is a simple React hello world code. It has an element with id root. We create elements via React library and we render them at root element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>React Hello World</title>
<script src="https://unpkg.com/react@18.2.0/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@18.2.0/umd/react-dom.production.min.js"></script>
</head>
<body>
<div id="root"></div>
<script>
const HelloWorld = () => {
return React.createElement(
'h1',
null, // no props
'Hello, World!' // content
);
};
const myReactElement = React.createElement(HelloWorld);
const rootElement = document.getElementById('root');
ReactDOM.render(myReactElement, rootElement);
</script>
</body>
</html>
Loading libraries react.production.min.js and react-dom.production.min.js is required for a any react project.
This code creates an element <h1> with no properties (null) and the content of ‘Hello, World!’; and this element is loaded at the root element.
React developer tools
If you use Firefox or Chrome, you can install React developer tools extension to help inspecting and debugging React web applications.
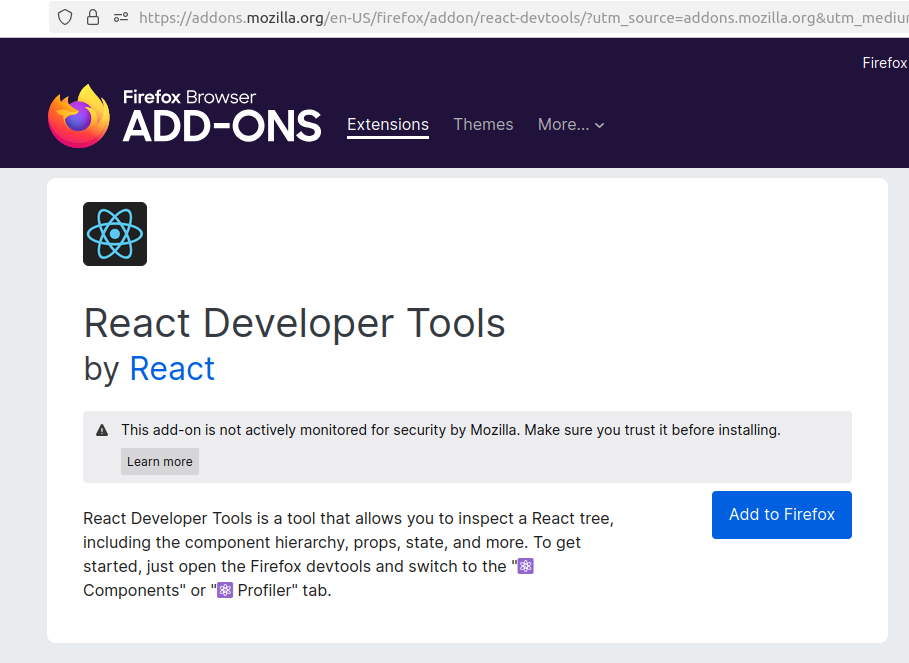
Then you will see new features at development tool such as components which shows you react element hierarchies on any React website and you can inspect them:
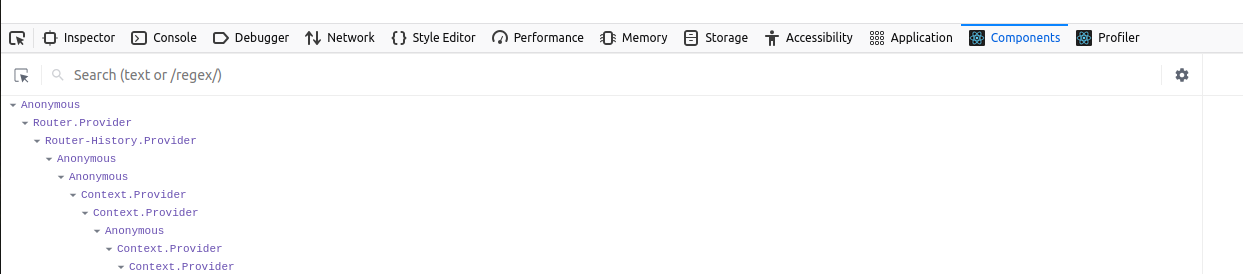
Properties in React and creating multi-child elements
React.createElement is not able to create multiple element but it can create multiple children, thus, we can place our multiple elements in a single wrapper div element.
Additionally, we previously passed null as a property. If you need to add HTML attributes, you can send them as React properties:
const Welcome = () => {
return React.createElement(
'div', // wrapper
{ // properties
style: {
backgroundColor: 'lightblue',
padding: '20px',
textAlign: 'center',
borderRadius: '10px'
}
},
React.createElement('h1', null, 'Welcome to React!'),
React.createElement('p', null, 'This is a simple example.'),
React.createElement('button', { onClick: () => alert('Button clicked!') }, 'Click Me')
);
};
ReactDOM.render(
React.createElement(Welcome),
document.getElementById('root')
);
This code will render to:

Using React with JSX
JSX (JavaScript XML) is a syntax extension for JavaScript commonly used with React. It allows developers to write HTML-like code directly within JavaScript.
To use JSX, you will need to load an additional library as follows:
<script src="https://unpkg.com/@babel/standalone"></script>
Then, you are allowed to write with JSX code in your React application as follows:
<script type="text/babel">
const Welcome = () => {
return (
<div
style={{
backgroundColor: 'lightblue',
padding: '20px',
textAlign: 'center',
borderRadius: '10px'
}}
>
<h1>Welcome to React!</h1>
<p>This is a simple example.</p>
<button onClick={() => alert('Button clicked!')}>Click Me</button>
</div>
);
};
ReactDOM.render(
<Welcome />,
document.getElementById('root')
);
</script>
Please note that inside <script type="text/babel">, the type set to text/babel so the script will be interpreted as JSX rather than standard Javascript.
Additionally, it is recommended that custom tags are name with first letter as capital so you anyone reading your code does not get confused between HTML tags and custom tags.
Passing properties
The string property values are wrapped inside a double quotation. While, other values are wrapped inside curly braces {} as follows
<script type="text/babel">
const Welcome = ({ title, isGreeting, count, items }) => {
return (
<div
style={{
backgroundColor: 'lightblue',
padding: '20px',
textAlign: 'center',
borderRadius: '10px'
}}
>
<h1>{title}</h1>
{isGreeting && <p>Hello! Welcome to React!</p>}
<p>You have {count} new notifications.</p>
<ul style={{
listStyleType: 'disc', // list-style-type
paddingLeft: 0, // padding-left
display: 'inline-block',
textAlign: 'left' // text-align
}}>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<div><button onClick={() => alert('Button clicked!')}>Click Me</button></div>
</div>
);
};
ReactDOM.render(
<Welcome
title="Welcome to React!"
isGreeting={true}
count={5}
items={['Item 1', 'Item 2', 'Item 3', 'Item 4']}
/>,
document.getElementById('root')
);
</script>
React fragments
As mentioned earlier, in React, ReactDOM.render would like to receive a single element to render. Thus, if we have multiple elements such as <Header /><Content /><Footer />, then we need to put them all under a wrapper such as <div> and pas it as a single element to get around this limit. This is not a big issue, nevertheless, we still have options to get around it by <React.Fragment> or even its short hand equivalent <> as follows:
const MyComponent = () => {
return (
<React.Fragment>
<h1>Title</h1>
<p>This is a paragraph.</p>
<button>Click Me</button>
</React.Fragment>
);
};
and the short syntax:
const MyComponent = () => {
return (
<>
<h1>Title</h1>
<p>This is a paragraph.</p>
<button>Click Me</button>
</>
);
};